Integrating live cricket scores into a WordPress website enhances user engagement, especially for sports-centric platforms. APIs (Application Programming Interfaces) streamline this process by fetching real-time data from reliable sources. This guide details methods to display live cricket scores on WordPress via API, including plugin-based solutions and custom coding approaches.
Understanding Cricket APIs
Cricket APIs provide structured data feeds for live scores, player statistics, match schedules, and historical records. Third-party providers like Cricketdata.org, CricAPI, and ESPNcricinfo offer RESTful APIs with JSON/XML responses.
Key Features of Cricket APIs:
- Real-time score updates
- Ball-by-ball commentary
- Player and team statistics
- Match schedules and results
Choosing a Cricket API
Select an API based on data accuracy, update frequency, and ease of integration. Below is a comparison of popular cricket APIs:
API Provider | Free Tier | Data Coverage | Documentation |
---|---|---|---|
Cricketdata.org | Yes | Global matches | Detailed |
CricAPI | Limited | International leagues | Moderate |
ESPNcricinfo | No | Comprehensive | Advanced |
For most WordPress users, Cricketdata.org offers a balance between reliability and accessibility.
Step 1: Obtain an API Key
- Sign up on the provider’s website (e.g., Cricketdata.org).
- Generate an API key from the dashboard.
- Note the endpoint URL for live scores (e.g.,
https://api.cricketdata.org/v1/scores
).
Step 2: Display Scores Using Plugins
Option A: SportsPress Plugin
SportsPress is a dedicated plugin for sports websites.
- Install and activate SportsPress.
- Navigate to SportsPress → Configure.
- Select “Cricket” as the default sport.
- Use the provided shortcode
[event_blocks]
to display live scores.
Option B: WP API Integration Plugin
For custom APIs, use WP API Integration:
- Install the plugin.
- Go to Settings → API Integration.
- Enter the API endpoint and authentication key.
- Use the shortcode
[api_data endpoint="scores"]
in posts/pages.
Step 3: Custom Code Integration
For developers, integrate the API directly into WordPress themes:
- Create a Custom Function:
Add this code tofunctions.php
:
PHP code
function fetch_live_scores() { $api_key = 'YOUR_API_KEY'; $endpoint = 'https://api.cricketdata.org/v1/scores'; $response = wp_remote_get( $endpoint, array( 'headers' => array( 'Authorization' => 'Bearer ' . $api_key ) )); if ( !is_wp_error( $response ) ) { $data = json_decode( $response['body'], true ); return $data; } }
- Display Scores in a Template:
Create a custom template (e.g.,live-scores.php
):
PHP code
<?php $scores = fetch_live_scores(); if ( $scores ) { echo '<div class="live-scores">'; foreach ( $scores as $match ) { echo '<div class="match">'; echo '<h3>' . $match['teams'] . '</h3>'; echo '<p>Score: ' . $match['score'] . '</p>'; echo '</div>'; } echo '</div>'; } ?>
- Style with CSS:
Add styling tostyle.css
:
CSS code
.live-scores { border: 1px solid #ddd; padding: 15px; } .match { margin-bottom: 10px; }
Custom Code to Display Live Cricket Scores on WordPress Via API
Your_theme/function.php — Add in the last.
function fetch_ipl_live_scores() {
$api_key = 'YOUR_API_KEY'; //change your API here
$response = wp_remote_get("https://api.cricapi.com/v1/currentMatches?apikey={$api_key}&offset=0");
if (is_wp_error($response) || 200 !== wp_remote_retrieve_response_code($response)) {
return '';
}
$data = json_decode(wp_remote_retrieve_body($response), true);
//var_dump($data);
//echo json_encode($data, JSON_PRETTY_PRINT);
if (empty($data['data']) || $data['status'] !== 'success') {
return '';
}
// Sort matches by date
$matches = $data['data'];
usort($matches, function($a, $b) {
$dateA = strtotime($a['date']);
$dateB = strtotime($b['date']);
return $dateB - $dateA; // Descending order
});
$output = '<div class="live-scores">';
foreach ($matches as $match) {
if (empty($match['teams']) || count($match['teams']) < 2) continue;
// Output structure remains the same as previous
$output .= sprintf(
'<div class="match">
<h3>%s</h3>
<div class="match-meta">
<p><strong>Date:</strong> %s</p>
<p><strong>Format:</strong> %s</p>
<p><strong>Venue:</strong> %s</p>
</div>',
esc_html($match['name']),
date('F j, Y', strtotime($match['date'])), // Formatted date
esc_html(strtoupper($match['matchType'])),
esc_html($match['venue'])
);
// Teams
$output .= '<div class="teams">';
foreach ($match['teams'] as $index => $team) {
$output .= sprintf(
'<div class="team team-%d">
<h4>Team %d:</h4>
<p>%s</p>
</div>',
$index + 1,
$index + 1,
esc_html($team)
);
}
$output .= '</div>';
// Scores
if (!empty($match['score'])) {
$output .= '<div class="scorecard"><h4>Scores:</h4>';
foreach ($match['score'] as $inning) {
$output .= sprintf(
'<div class="inning">
<p><strong>%s:</strong> %d/%d (%s overs)</p>
</div>',
esc_html($inning['inning']),
esc_html($inning['r']),
esc_html($inning['w']),
esc_html($inning['o'])
);
}
$output .= '</div>';
}
// Match status
$output .= sprintf(
'<div class="status">
<p><strong>Status:</strong> %s</p>
<p>Match %s</p>
</div>',
esc_html($match['status']),
$match['matchEnded'] ? 'Concluded' : 'In Progress'
);
$output .= '</div>'; // Close match div
}
$output .= '</div>';
return !empty(strip_tags($output)) ? $output : '';
}
add_shortcode('ipl_live_scores', 'fetch_ipl_live_scores');
Then, create a new page or post and insert this short code.
[ipl_live_scores]
Go to theme customize settings and add this CSS
/* Add this to your theme's CSS */
.live-scores {
font-family: Arial, sans-serif;
max-width: 800px;
margin: 0 auto;
}
.match {
border: 1px solid #ddd;
padding: 20px;
margin-bottom: 20px;
border-radius: 8px;
}
.match-meta p {
margin: 5px 0;
color: #666;
}
.teams {
display: flex;
gap: 30px;
margin: 15px 0;
}
.scorecard {
background: #f5f5f5;
padding: 15px;
border-radius: 5px;
margin: 10px 0;
}
.status {
color: #2c3e50;
font-weight: bold;
margin-top: 10px;
}
Check the Output here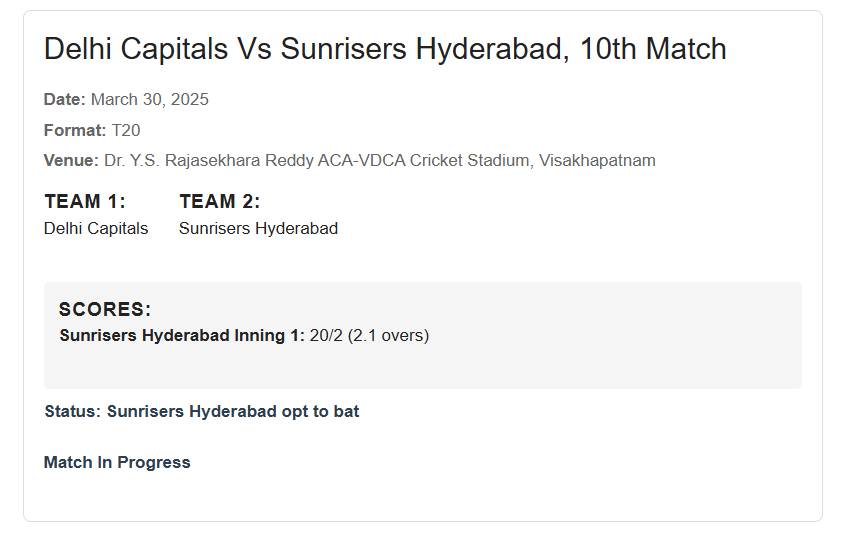
Optimizing Live Score Display
- Caching: Use WP Rocket to cache API responses and reduce server load.
- Mobile Responsiveness: Ensure themes like GeneratePress adapt scores for mobile users.
- SEO: Apply on-page SEO techniques to score widgets for better indexing.
Troubleshooting Common Issues
Issue | Solution |
---|---|
API data not loading | Check API key validity and endpoint URL |
Slow page load times | Enable caching or reduce API call frequency |
Formatting errors | Validate JSON responses and CSS conflicts |
Enhancing User Engagement
- Add push notifications using PushEngage.
- Integrate live score widgets with social media for cross-platform updates.